Kết nối android với mysql (Phần 3)
Bài viết kết nối android với mysql (phần 3) sẽ hướng dẫn các bạn sử dụng REST API trong Android sử dụng PHP, Slim và MySQL. Các thao tác thêm và truy vấn dữ liệu MySQL từ ứng dụng Android cũng sẽ được trình bày trong bài viết này.
Chương trình được xây dựng trong bài viết này gồm 3 màn hình. Màn hình thứ nhất gồm 2 nút cho phép người dùng lựa chọn chức năng thêm mới tài khoản hoặc chức năng đăng nhập vào hệ thống.
Màn hình chính

Màn hình thêm mới tài khoản

Màn hình đăng nhập vào hệ thống

Kết nối android với mysql – Chuẩn bị
1. REST API
3. Icon
Kết nối android với mysql – Các bước thực hiện
Bước 1: Cài đặt webserver XAMPP. Trường hợp đã cài đặt webserver thì bỏ qua bước này
Bước 2: Tạo thư mục rest trong thư mục htdocs của XAMPP -> sau đó giải nén tập tin rest.rar vào thư mục rest (chi tiết vui lòng xem hình bên dưới)

Bước 3: Truy cập http://localhost:port/phpmyadmin và tạo cơ sở dữ liệu
Chọn Import -> chọn Choose File, chọn tập tin rest.sql -> chọn Go

Bước 4: Thiết kế giao diện và viết xử lý
Tạo lớp MyService
import android.util.Log;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URLEncodedUtils;
import org.apache.http.impl.client.DefaultHttpClient;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.util.List;
/**
* Created by GiaSuTinHoc.vn on 28/12/2015.
*/
public class MyService {
static InputStream is = null;
static String response = null;
public final static int GET = 1;
public final static int POST = 2;
public String makeService(String url, int method) {
return this.makeService(url, method, null);
}
public String makeService(String url, int method, List<NameValuePair> params) {
try {
// http client
DefaultHttpClient httpClient = new DefaultHttpClient();
HttpEntity httpEntity = null;
HttpResponse httpResponse = null;
// Checking http request method type
if (method == POST) {
HttpPost httpPost = new HttpPost(url);
// adding post params
if (params != null) {
httpPost.setEntity(new UrlEncodedFormEntity(params));
}
httpResponse = httpClient.execute(httpPost);
} else if (method == GET) {
// appending params to url
if (params != null) {
String paramString = URLEncodedUtils.format(params, "utf-8");
url += "?" + paramString;
}
HttpGet httpGet = new HttpGet(url);
httpResponse = httpClient.execute(httpGet);
}
httpEntity = httpResponse.getEntity();
is = httpEntity.getContent();
} catch (Exception ex) {
Log.e("Buffer Error", "Error: " + e.toString());
}
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(is, "UTF-8"), 8);
StringBuilder sb = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
sb.append(line + "\n");
}
is.close();
response = sb.toString();
} catch (Exception e) {
Log.e("Buffer Error", "Error: " + e.toString());
}
return response;
}
}
Tạo activity tên RestRegisterActivity

Viết xử lý
import android.androidpractice.mysql.MyService; import android.app.NotificationManager; import android.app.ProgressDialog; import android.content.Context; import android.os.AsyncTask; import android.support.v7.app.AppCompatActivity; import android.support.v4.app.NotificationCompat; import android.support.v4.app.NotificationCompat.Builder; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.EditText; import org.apache.http.NameValuePair; import org.apache.http.message.BasicNameValuePair; import org.json.JSONException; import org.json.JSONObject; import java.util.ArrayList; import java.util.List; public class RestRegisterActivity extends AppCompatActivity { ProgressDialog pd; MyService sh; final String URL_REGISTER = "http://10.0.2.2:port/rest/register"; EditText etName, etEmail, etPassword; private NotificationManager nm; private Builder b; int id = 1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_rest_register); etName = (EditText)findViewById(R.id.etName); etEmail = (EditText)findViewById(R.id.etEmail); etPassword = (EditText)findViewById(R.id.etPassword); sh = new MyService(); nm = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); b = new NotificationCompat.Builder(RestRegisterActivity.this); } public void registerAction(View view){ String name, email, password; name = etName.getText().toString(); email = etEmail.getText().toString(); password = etPassword.getText().toString(); new RegisterWS(name,email, password).execute(); } private void createNotification(String contentTitle, String contentText, String error) { b.setContentTitle(contentTitle) b.setContentText(contentText); if("false".equals(error)) b.setSmallIcon(R.drawable.icon_success); else b.setSmallIcon(R.drawable.ic_error); nm.notify(id, b.build()); } //inner class class RegisterWS extends AsyncTask { String name; String email; String password; public RegisterWS(String name, String email, String password) { this.name = name; this.email = email; this.password = password; } @Override protected void onPreExecute() { super.onPreExecute(); pd = new ProgressDialog(RestRegisterActivity.this); pd.setMessage("Creating..."); pd.setIndeterminate(false); pd.setCancelable(true); pd.show(); } @Override protected Object doInBackground(Object[] params) { // Building Parameters List<NameValuePair> args = new ArrayList<NameValuePair>(); args.add(new BasicNameValuePair("name", name)); args.add(new BasicNameValuePair("email", email)); args.add(new BasicNameValuePair("password", password)); // getting JSON Object String json = sh.makeService(URL_REGISTER, MyService.POST, args); if (json != null) { try { JSONObject jsonObject = new JSONObject(json); String error = jsonObject.getString("error"); if(!error.isEmpty()){ createNotification("Notification", jsonObject.getString("message"), error); } } catch (JSONException e) { Log.d("Error...", e.toString()); } } return null; } @Override protected void onPostExecute(Object o) { super.onPostExecute(o); pd.dismiss(); } } }
Tạo activity tên RestLoginActivity

Viết xử lý
import android.androidpractice.mysql.MyService; import android.app.NotificationManager; import android.app.ProgressDialog; import android.content.Context; import android.os.AsyncTask; import android.support.v4.app.NotificationCompat; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.EditText; import org.apache.http.NameValuePair; import org.apache.http.message.BasicNameValuePair; import org.json.JSONException; import org.json.JSONObject; import java.util.ArrayList; import java.util.List; public class RestLoginActivity extends AppCompatActivity { ProgressDialog pd; MyService sh; final String URL_LOGIN = "http://10.0.2.2:port/rest/login"; EditText etEmail, etPassword; private NotificationManager nm; private NotificationCompat.Builder b; int id = 1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_rest_login); etEmail = (EditText)findViewById(R.id.etEmail_Login); etPassword = (EditText)findViewById(R.id.etPassword_Login); sh = new MyService(); nm = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); b = new NotificationCompat.Builder(RestLoginActivity.this); } public void loginAction(View view){ String email, password; email = etEmail.getText().toString(); password = etPassword.getText().toString(); new WS(email, password).execute(); } private void createNotification(String contentTitle, String contentText, String error) { b.setContentTitle(contentTitle); b.setContentText(contentText); if("false".equals(error)) { b.setSmallIcon(R.drawable.icon_success); } else { b.setSmallIcon(R.drawable.ic_error); } nm.notify(id, b.build()); } //inner class class WS extends AsyncTask { String email; String password; public WS(String email, String password) { this.email = email; this.password = password; } @Override protected void onPreExecute() { super.onPreExecute(); pd = new ProgressDialog(RestLoginActivity.this); pd.setMessage("Handling..."); pd.setIndeterminate(false); pd.setCancelable(true); pd.show(); } @Override protected Object doInBackground(Object[] params) { // Building Parameters List<NameValuePair> args = new ArrayList<NameValuePair>(); args.add(new BasicNameValuePair("email", email)); args.add(new BasicNameValuePair("password", password)); // getting JSON Object String json = sh.makeServiceCall(URL_LOGIN, MyService.POST, args); if (json != null) { try { JSONObject jsonObject = new JSONObject(json); String error = jsonObject.getString("error"); if("true".equals(error)){ createNotification("Notification", jsonObject.getString("message"), error); } else { createNotification("Notification", "Login successful", error); } } catch (JSONException e) { Log.d("Error...", e.toString()); } } return null; } @Override protected void onPostExecute(Object o) { super.onPostExecute(o); pd.dismiss(); } } }
Tạo activity tên MainRestActivity

Viết xử lý
import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; public class MainRestActivity extends AppCompatActivity { Intent intent; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main_rest); } public void registerActivity(View view){ intent = new Intent(this, RestRegisterActivity.class); startActivity(intent); } public void loginActivity(View view){ intent = new Intent(this, RestLoginActivity.class); startActivity(intent); } }
Kết nối android với mysql – Build và run
Màn hình đăng ký
Trường hợp không nhập thông tin và chạm nút REGISTER
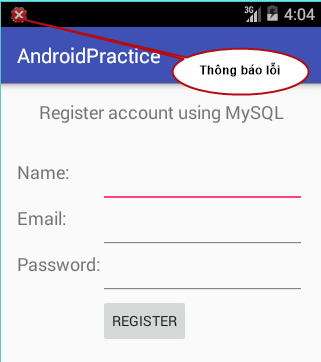
Chạm, giữ và di chuyển về phía bên dưới để xem thông báo

Trường hợp nhập đầy đủ thông tin và chạm vào nút REGISTER


Chạm, giữ và di chuyển về phía bên dưới để xem thông báo
Màn hình đăng nhập
Trường hợp không nhập thông tin và chạm vào nút LOGIN

Thông báo lỗi

Trường hợp nhập đầy đủ thông tin và hợp lệ

Xem thông báo
